Bring your favorite React frontend
Cosmic and React are a perfect combination.
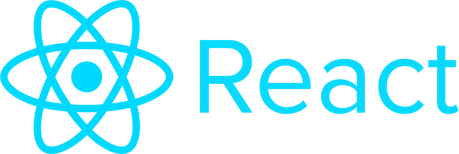
Why use a Headless CMS as React’s CMS?
Using a headless CMS for your React application eliminates cumbersome layers of technological setup and maintenance from your React CMS that are necessary for coupled and decoupled CMS systems (e.g. WordPress, Drupal, Joomla, Shopify, Magento, etc.) Headless CMS data is accessible and extensible, providing future-proofed delivery via API to any destination. This circumvents investing in CMS infrastructure maintenance, allowing development focus to be on presentation and application business logic.
Cosmic is the Best Headless CMS for React
Cosmic's headless CMS makes it a breeze to manage and deliver React CMS content for websites, applications, or platforms. Our feature set is unmatched - it's our goal to provide a product that eliminates your need to work on CMS infrastructure. We also provide pre-built examples so you can kick-start your projects, or facilitate new purposes and use cases. What's more, our React resources and documentation are unparalleled.
With Cosmic, developers build better React applications, faster
Top-Tier React CMS Features
Cosmic offers world-class API speed, industry-leading uptime reports, and a verbose feature set built for React.
- Component composition with Objects
- Media / file management
- Easy access to documentation and code samples
- Extensive NPM module
- and more
React CMS templates
Use one of our starter templates to start building your own React solution today.
Agency Website
Simple Next.js Blog
Developer Portfolio
Resources
React resources
One thing that sets us apart is our extensive developer resources. Check out some of our favorite articles and videos below, learn more about what to look for in a good React CMS, and how we've made Cosmic best-in-class.
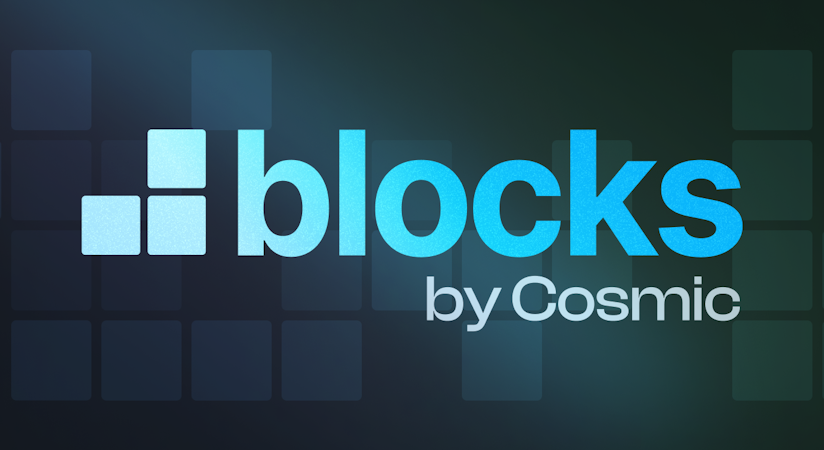
Introducing Blocks
Cosmic
January 23, 2024
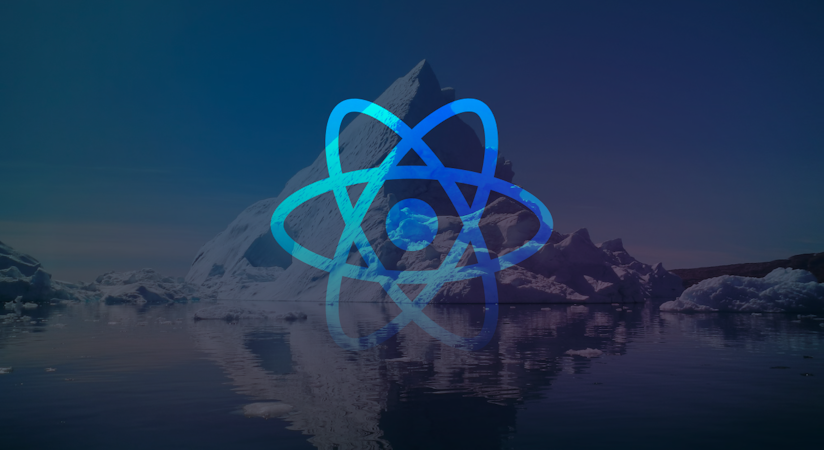
Best Practices using React Server Components with a Headless CMS
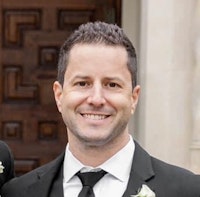
Tony Spiro
December 05, 2023
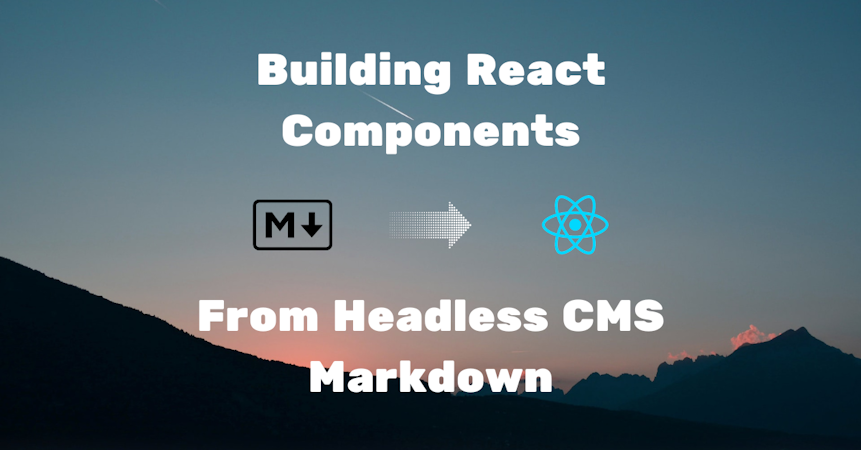
Building React Components from headless CMS markdown
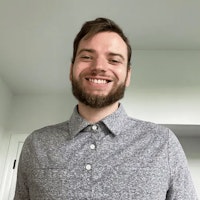
Stefan Kudla
August 24, 2022
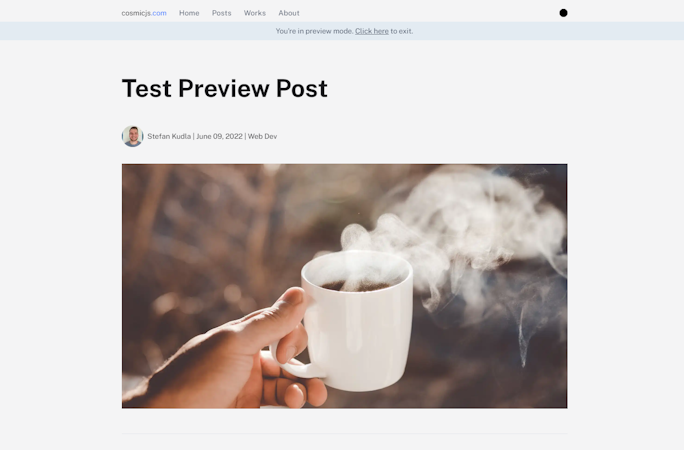
How to use Next.js Preview Mode with the Cosmic headless CMS
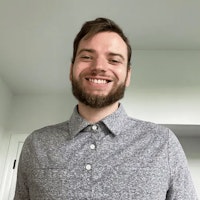
Stefan Kudla
August 09, 2022
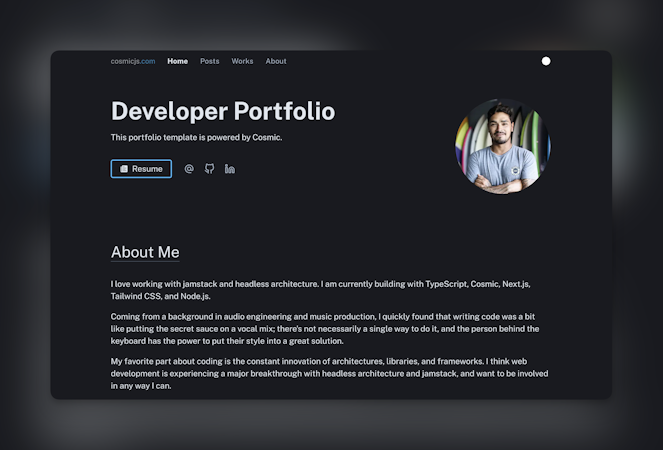
Creating a Developer Portfolio with Next.js and Cosmic
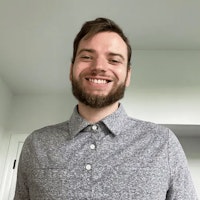
Stefan Kudla
July 19, 2022
Uptime you can depend on
Reliable infrastructure
No need to fear downtime for your content. Our service is built to auto scale through bursts of high traffic so you can focus on your content and business, we'll handle the infrastructure.
Upgrade your uptime
Get a 99.95% uptime guarantee with our Enterprise plans.
Trust our history
Check out our status page for historical uptime on our serverless, cloud infrastructure.